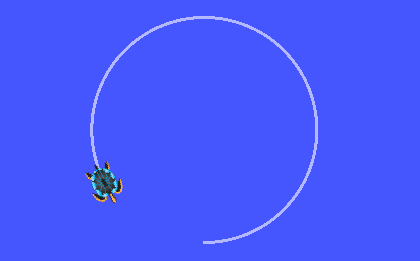
ROS-Publisher
Study note for ROS publisher.
Model of Topic
- Publisher(turtle Velocity)
- –[Message(geometry_msgs::Twist)]–>
- Topic (/turtle1/cmd_vel)
- –[Message(geometry_msgs::Twist)]–>
- Subscriber(turtlesim)
Programming realization
Take turtlesim as the example.
We write a program acting like a publisher and sending controlling messages.
Create package
1 | $ cd catkin_ws/src |
can see:
1 | $ ls |
How to realize a publisher?
- Initialize ROS node
- register node info from ROS Master, including name of the topic and msg type
- create message data
- publish message at a specific frequency
Code
create velocity_publisher.cpp
file in learning_topic/src
1 |
|
What is queue length?
- msgs can be published very fast while the data transmission rate is limited, so the queue act as a buffer
- first in, first thrown, keeping the remained msgs in the queue relatively new
Configure the compile rule of publisher
Steps:
- set the code need to be complied and the generated excutable file
- set the link library
Add two lines to learning\_topic/CMakeLists.txt
(in build part):
1 | add_executable(velocity_publisher src/velocity_publisher.cpp) |
Compile
1 | $ cd ~/ROS_study/catkin_ws |
Then, you can see turtle:
And outputs:
1 | ... |